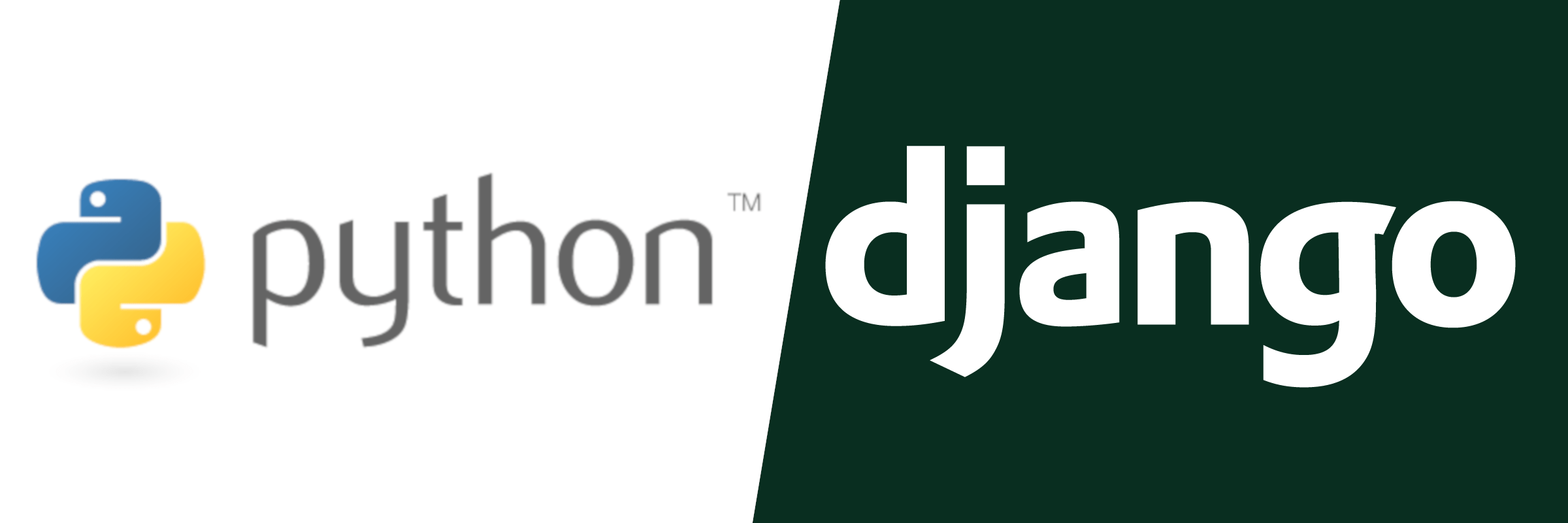
Recently, I’m digging into python Django framework to practice python and to learn a skill when I quit my job as a project manager _(or get fired)_. So here are some notes that I’ve put together that helps me understand what and which I should do. It’s also a flow that I follow every time learning a new framework in any programming language.
settings.py and any other configurations
Get familiar with the settings and configs would save you a lot of efforts.
Whenever you found a function/feature that is missing in the framework you’re learning, don’t panic. First looking into the settings and its documentation, which you sometimes found out the author of this framework has already thought of this and put them in the settings as an option for you to enable/disable.
I guess the reason why they (the authors) doing this is because they don’t want to spook you with tens of thousands of settings, switches, unknown file paths, and API keys and such.The first line of codes I'm looking at while learning Django
1
2
3
4
5
6
7
8
9
10DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql', # 数据库引擎
'NAME': 'mydb', # 你要存储数据的库名,事先要创建之
'USER': 'root', # 数据库用户名
'PASSWORD': '1234', # 密码
'HOST': 'localhost', # 主机
'PORT': '3306', # 数据库使用的端口
}
}
Database migration
Why I’m looking into this is because I had another scrapy
project that scraped tons of things into the database, as back then I don’t know what I would like to do with the information I scraped.
Now I’m learning this frontend framework _(it’s not accurate since Django also has backend/admin to work with)_, I would like to use the existing DB and data inside it instead of building db and dumping data from scratch. Also this would make migrating project to another project easier, saving more disk space as I’m working mostly my projects on my local machine.
Connecting existing mysql DB
python3不支持MySQLdb,可用pymysql代替。cmd安装pymysql:pip install pymysql。
在项目文件夹下的_init_.py添加如下代码即可。
1 | import pymysql |
然后在Terminal中执行数据库迁移命令:1
2python manage.py makemigrations
python manage.py migrate
To dump the data tables creation into SQL. The exact output depends on the database you are using1
python3 manage.py sqlmigrate timehutBlog 0001
- Changing DATABASE setting in
settings.py
- Use
python3 manage.py inspectdb > models.py
to generate Mysql schema - Modify models.py schema
python3 manage.py migrate
Creating an administration site for your models
Creating a superuser
1 | python3 manage.py createsuperuser |
Then access http://127.0.0.1:8000/admin/
Sharing posts by e-mail
Just one feature that I think is common so that I saved the information here for future uses.
- Create a form for users to fill in their name and e-mail, the e-mail recipient, and optional comments
- Form: Allows you to build standard forms
- ModelForm: Allows you to build forms to create or update model instances
1
2Note
Forms can reside anywhere in your Django project but the convention is to place them inside a forms.py file for each application.
- Create a view in the
views.py
file that handles the posted data and sends the e-mail- external SMTP server by adding the following settings in the settings.py file of your project:
1
2
3
4
5
6EMAIL_HOST: The SMTP server host. Default localhost.
EMAIL_PORT: The SMTP port Default 25.
EMAIL_HOST_USER: Username for the SMTP server.
EMAIL_HOST_PASSWORD: Password for the SMTP server.
EMAIL_USE_TLS: Whether to use a TLS secure connection.
EMAIL_USE_SSL: Whether to use an implicit TLS secure connection.”
- external SMTP server by adding the following settings in the settings.py file of your project:
- Add an URL pattern for the new view in the
urls.py
file of the blog application - Create a template to display the form
Create comment system
Reason same as above feature.
- Create models.py
- Create form
- Remember that Django has two base classes to build forms: Form and ModelForm.
- Handling ModelForms in views
- save() is available for ModelForm but not for Form instance, since they are not linked to any model
- Adding comments to the collection detail template
- Display the total number of comments for the post
- Display the list of comments
- Display a form for users tp add a new comment
Extending Your Blog Application
Feature for searching
- Creating custom template tags and filters
- Adding a sitemap and a post feed
- Building a search enging with Solr and Haystack
Creating custom template tags and filters
Custom template tags and filters are extremely important and you need to be able to get yourself familiar with. As Django using built-in template/tags/filters to render the variables either from your backend API or from DB, to make sure you’re able to customize the data to the format that you need is very crucial.
- Creating custom template tags
simple_tag
: Processes the data and returns a stringinclusion_tag
: Processes the data and returns a rendered templateassignment_tag
: Processes the data and sets a variable in the context (assignment_tag
is depricated in Django 2.0)
‘’’
Template tags must live inside Django applications
‘’’
- Creating custom template filters
Adding a sitemap to your site
Just for notes
in sitemap.py1
from django.contrib.sitemaps import Sitemap
in urls.py1
2
3
4
5
6
7
8
9from django.contrib.sitemaps.views import sitemap
from blog.sitemaps import PostSitemap
...
...
urlpatterns = [
...
...
path('sitemap.xml', sitemap, {'sitemaps': sitemaps}, name='django.contrib.sitemaps.views.sitemap')
]
Adding a RSS feed
Just for notes
in feeds.py1
2
3from django.contrib.syndication.views import Feed
...
...